Xceed Grid for WinForms provides a variety of rows which can be added to sections of a grid or group. These rows can be used to display information such as labels, manage other rows in the grid, insert new data rows, etc.
Adding rows
Every row, with the exception of the DataRow, can be added to the header and footer sections of a grid or group. For example, to add an InsertionRow to the fixed header section of the grid, the following code would be used:
C# |
Copy Code |
gridControl1.FixedHeaderRows.Add( new InsertionRow() );
|
When using the Grid Designer, rows can be added by right clicking on any element in the grid and selecting the Add row... or Insert row... menu. It is also possible to add rows using the verbs in the property grid.
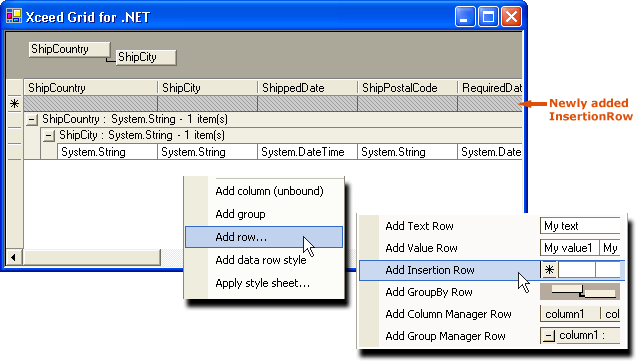
Adding DataRows
DataRows can also be added to the grid however they can only be added to the grid's body when the grid is not bound to a data source.
To add DataRows to the grid, the AddNew method of the grid's DataRows property must be used. The row's data can then be provided immediately or by handling the AddingDataRow event. The desired number of unbound columns must be added prior to adding the data rows to the grid. For example:
C# |
Copy Code |
// Add the desired number of columns to the grid. In the case, we will only add 4 gridControl1.Columns.Add( new Column( "column1", typeof( int ) ) ); gridControl1.Columns.Add( new Column( "column2", typeof( int ) ) ); gridControl1.Columns.Add( new Column( "column3", typeof( int ) ) ); gridControl1.Columns.Add( new Column( "column4", typeof( int ) ) );
// Subscribe to the AddingDataRow event gridControl1.AddingDataRow += new AddingDataRowEventHandler( grid_AddingDataRow );
// Add the desired number of DataRow objects to the grid's collection of datarows for( int i = 0; i < 50; i++ ) { gridControl1.DataRows.AddNew().EndEdit();
// AddNew will return a reference to a new DataRow object whose // cell values we can fill immediately, for the purposes of this // example we will use the AddingDataRow event instead. // // Xceed.Grid.DataRow row = gridControl1.DataRows.AddNew(); // row.Cells[ 0 ].Value = 25; // row.Cells[ 1 ].Value = 26; // row.Cells[ 2 ].Value = 27; // row.Cells[ 3 ].Value = 28; // row.EndEdit(); // etc. }
// Private member variables that will be used to provide data to the grid. private Random m_random = new Random();
// This is the procedure that will handle the AddingDataRow event. private void grid_AddingDataRow( object sender, AddingDataRowEventArgs e ) { try { foreach( Cell cell in e.DataRow.Cells ) { cell.Value = m_random.Next( 0, 5000 ); } } catch( Exception exception ) { MessageBox.Show( exception.ToString() ); } }
|
Make sure that you call the EndEdit method after calling AddNew in order to add the newly created datarow to the grid. Note that if EndEdit is not called and another call to AddNew is made, the previous row created with AddNew will be added to the grid.