The SurfaceSeriesBase class is the base class for the GridSurfaceSeries and MeshSurfaceSeries classes, which represent two types of surface series. It is derived from SeriesBase and inherits all its functionality. The class extends it with common functionality for the Surface series, such as surface palette management, control of the surface filling style, frame style and appearance, flat surface mode, etc.
Surface Frame
The SurfaceSeriesBase object supports several frame styles including Mesh, Contour, Mesh Contour, Dots, and no frame. You can change the style of the frame with the FrameStyle property, which is of type SurfaceFrameStyle. The following code changes the frame style to mesh contour:
VB.NET |
|
surface.FrameStyle = SurfaceFrameStyle.MeshContour |
C# |
|
surface.FrameStyle = SurfaceFrameStyle.MeshContour; |
The frame can be rendered with uniform coloring or with palette coloring. This feature is controlled by the boolean property PaletteFrame. If it is turned off the surface frame is displayed with the color defined by the FrameLine properties. Otherwise, the frame is displayed with the current palette colors. The following images demonstrate the different frame styles.
Surface Filling
Surface series support three surface fill styles: Zone filling, Uniform filling, and no filling. The fill style is controlled with the FillStyle property of the SurfaceSeriesBase class. By default the filling mode is Zone: the surface is filled with the colors of the current palette, based on the elevation of the surface segments. The following code disables the filling.
VB.NET |
|
surface.FillStyle = SurfaceFillStyle.None |
C# |
|
surface.FillStyle = SurfaceFillStyle.None; |
The following image displays a typical zone-filled surface chart.
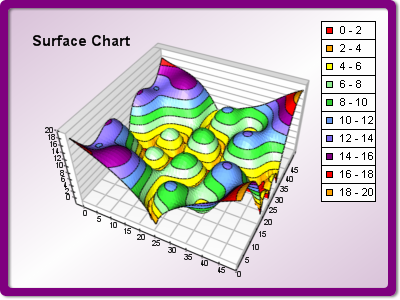
The FillEffect property gives access to a FillEffect object, with which you can control the surface transparency, apply gradients, images, patterns, and advanced gradients to the surface, as well as modify material properties like emissive color, specular reflection color, and shininess. The fill effect is merged with the palette colors. If you need to show a surface with some kind of fill effect, but without zoning (for example, an image mapped on the surface), you have to use Uniform filling mode:
VB.NET |
|
surface.FillStyle = SurfaceFillStyle.Uniform |
C# |
|
surface.FillStyle = SurfaceFillStyle.Uniform; |
Now you can apply the desired image with the help of the surface fill effect.
VB.NET |
|
surface.FillEffect.SetImage("c:\texture.png") |
C# |
|
surface.FillEffect.SetImage("c:\\texture.png"); |
The image below shows a surface with a mapped texture image:
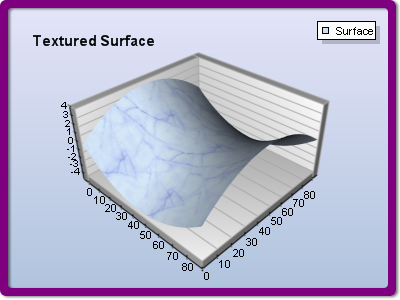
Surface Palette
The surface palette defines the colors for the surface filling (when SurfaceFillStyle.Zone is used), as well as the colors for the surface frame when the PaletteFrame property is set to true. By default the surface uses an automatically generated palette. The automatic palette can either be synchronized with the axis ticks, or a fixed number of palette steps can be defined. The mode is controlled by the boolean property SyncPaletteWithAxisScale. The number of steps is defined by the PaletteSteps property. The following code creates a palette with 4 steps:
VB.NET |
|
surface.SyncPaletteWithAxisScale = False surface.PaletteSteps = 4
|
C# |
|
surface.SyncPaletteWithAxisScale = false; surface.PaletteSteps = 4;
|
The advantage of these types of palette filling is that the component will automatically assign colors to values. Sometimes though, you might want to specify exactly the color mapped on a particular elevation. In this case, you can manually set the palette entries. The first step to take is to turn off the automatic palette.
VB.NET |
|
surface.AutomaticPalette = False |
C# |
|
surface.AutomaticPalette = false; |
The Palette property of the SurfaceSeriesBase returns an Palette object that helps you deal with palette entries. The Palette object is a set of elevation values and their corresponding color entries. The following code adds three entries to a palette:
VB.NET |
|
surface.Palette.Clear() surface.Palette.Add(10.0, Color.Red) surface.Palette.Add(20.5, Color.Green) surface.Palette.Add(40.0, Color.Blue)
|
C# |
|
surface.Palette.Clear(); surface.Palette.Add(10.0, Color.Red); surface.Palette.Add(20.5, Color.Green); surface.Palette.Add(40.0, Color.Blue);
|
The newly created palette will map red to value 10.0, green to value 20.5, and blue to value 40.0. As you can see, one of the advantages of the custom palette is that it can map colors to irregular value intervals. Another useful feature of the surface palette is smooth palette mode. In this mode the zone colors gradate smoothly from one to another. The following code turns on smooth palette mode.
VB.NET |
|
surface.SmoothPalette = True |
C# |
|
surface.SmoothPalette = true; |
The following images demonstrate a custom palette with smooth mode disabled and enabled.
Smooth and Zoned Surface Palette |
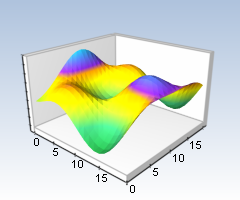 |
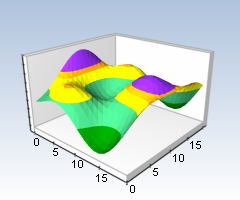 |
Contour Chart
The surface series can be displayed in flat surface mode (also known as contour chart). This mode is enabled with the DrawFlat property of the SurfaceSeriesBase class.
VB.NET |
|
surface.DrawFlat = True |
C# |
|
surface.DrawFlat = true; |
The user can position the contour at an arbitrary vertical position. Two properties control the vertical position of the contour: PositionMode and PositionValue. The contour can be positioned in three modes: SurfacePositionMode.AxisBegin, SurfacePositionMode.AxisEnd and SurfacePositionMode.CustomValue. When you set the PositionMode property to AxisBegin or AxisEnd the component ignores PositionValue and places the contour respectively at the min or the max value of the vertical axis. When you set PositionMode to CustomValue, the contour is positioned at the specified value from PositionValue. The following code positions the surface at value 5.0:
VB.NET |
|
surface.PositionMode = SurfacePositionMode.CustomValue surface.PositionValue = 5.0
|
C# |
|
surface.PositionMode = SurfacePositionMode.CustomValue; surface.PositionValue = 5.0;
|
The following images represent contour charts with smooth and zoned palettes.
Contour with Smooth and Zone Palette |
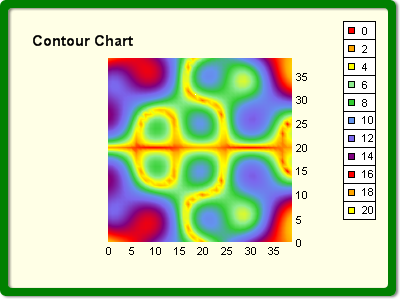 |
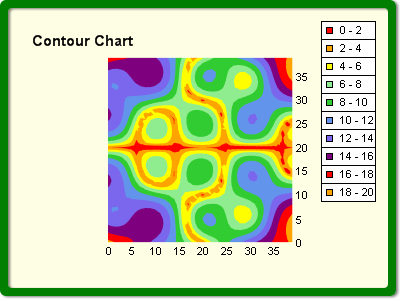 |
Contour charts are not usually displayed in perspective view, but rather with orthogonal projection in top-view position. This can easily be done with a special predefined projection. The following line of code demonstrates:
VB.NET |
|
chart.View.SetPredefinedProjection(PredefinedProjection.OrthogonalTop) |
C# |
|
chart.View.SetPredefinedProjection(PredefinedProjection.OrthogonalTop); |
Formatting of Surface Series
The surface series supports the following formatting commands for the legend's item texts:
<zonevalue> - Palette value (for smooth palette only).
<zonebegin> - Begin value of a zone (the lower value).
<zoneend> - Second value of a zone (the higher value).
The following formatting commands are supported for the tooltip texts:
<value> - Elevation value of a data point.
<index> - Index of a surface segment.
<xindex> - X index of a surface segment.
<zindex> - Z index of a surface segment.
The ValueFormatting property returns an ValueFormatting object, which defines the formatting properties for all elevation values.
Surface Series Palette Legend
The surface series populates the legend with palette information when the legend mode is SeriesLegendMode.SeriesCustom. If a smooth palette is applied, all the palette entries are displayed in the legend. The formatting command that should be used in such case is <zonevalue>.
VB.NET |
|
surface.Legend.Format = "<zonevalue>" |
C# |
|
surface.Legend.Format = "<zonevalue>"; |
If the smooth palette is turned off, the legend displays the zones defined by two successive palette entries. The number of legend items in this case is equal to the number of palette entries minus one. Use the following formatting commands: <zonebegin> for the first value in a zone, <zoneend> for the second value in a zone.
VB.NET |
|
surface.Legend.Format = "<zonebegin> - <zoneend>" |
C# |
|
surface.Legend.Format = "<zonebegin> - <zoneend>"; |
Surface Series Interactivity
The Interactivity property gives access to an SurfaceSeriesInteractivity object, which controls the interactivity features of the surface series. The following code enables the tooltips and sets up the interactivity object to show elevation values when the mouse is over a surface segment.
VB.NET |
|
chartControl.InteractivityOperations.Add(New TooltipInteractivityOperation()) surface.Interactivity.TooltipMode = SeriesTooltipMode.Formatted surface.Interactivity.TooltipFormat = "<value>" surface.ValueFormat.Format = ValueFormat.Custom surface.ValueFormat.CustomFormat = "0.00"
|
C# |
|
chartControl.InteractivityOperations.Add(new TooltipInteractivityOperation()); surface.Interactivity.TooltipMode = SeriesTooltipMode.Formatted; surface.Interactivity.TooltipFormat = "<value>"; surface.ValueFormat.Format = ValueFormat.Custom; surface.ValueFormat.CustomFormat = "0.00";
|
See Also
SurfaceSeriesBase | MeshSurfaceSeries | GridSurfaceSeries | Grid Surface | Mesh Surface